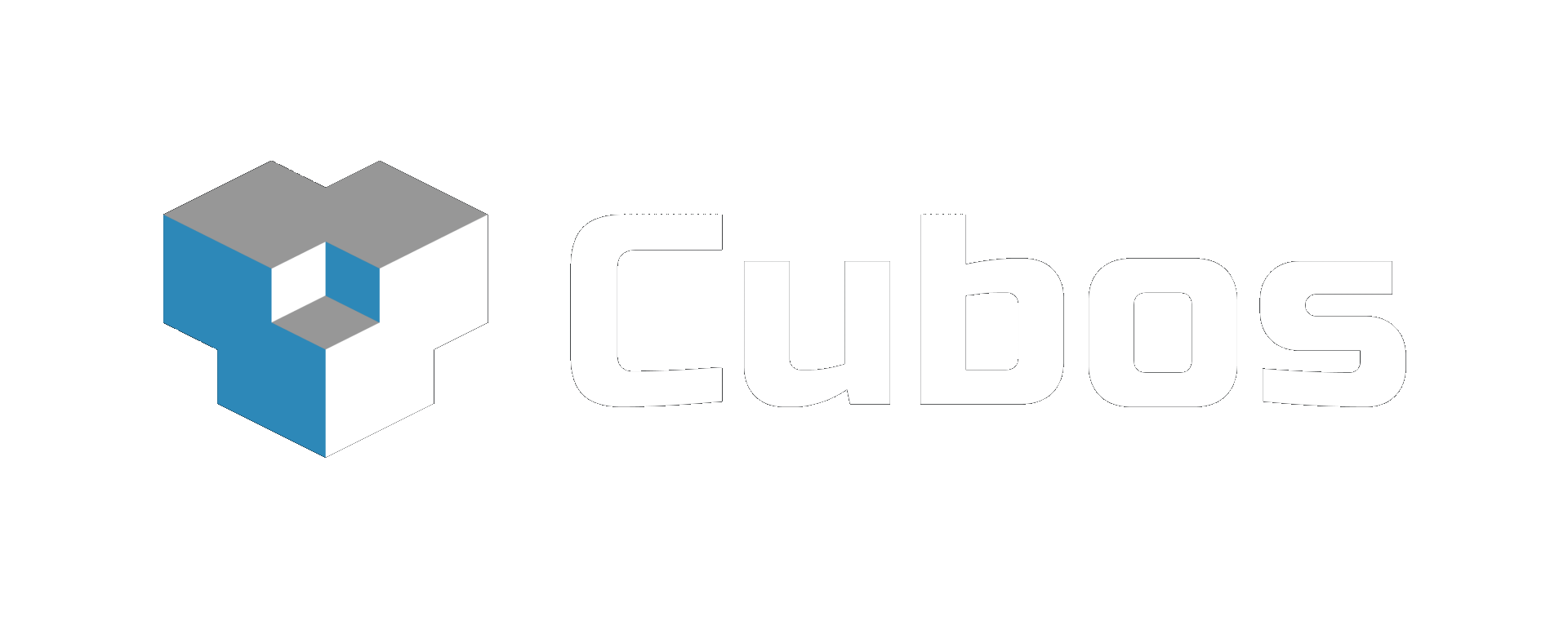
An open-source student-developed game engine built in modern C++ where everything is made of voxels.
Voxels First
Voxels are a first class citizen in Cubos: our engine comes with many plugins built specifically to enable you to create voxel games.
Data Oriented Design
Cubos is built on top of our own custom Entity Component System, designed both for performance and flexibility.
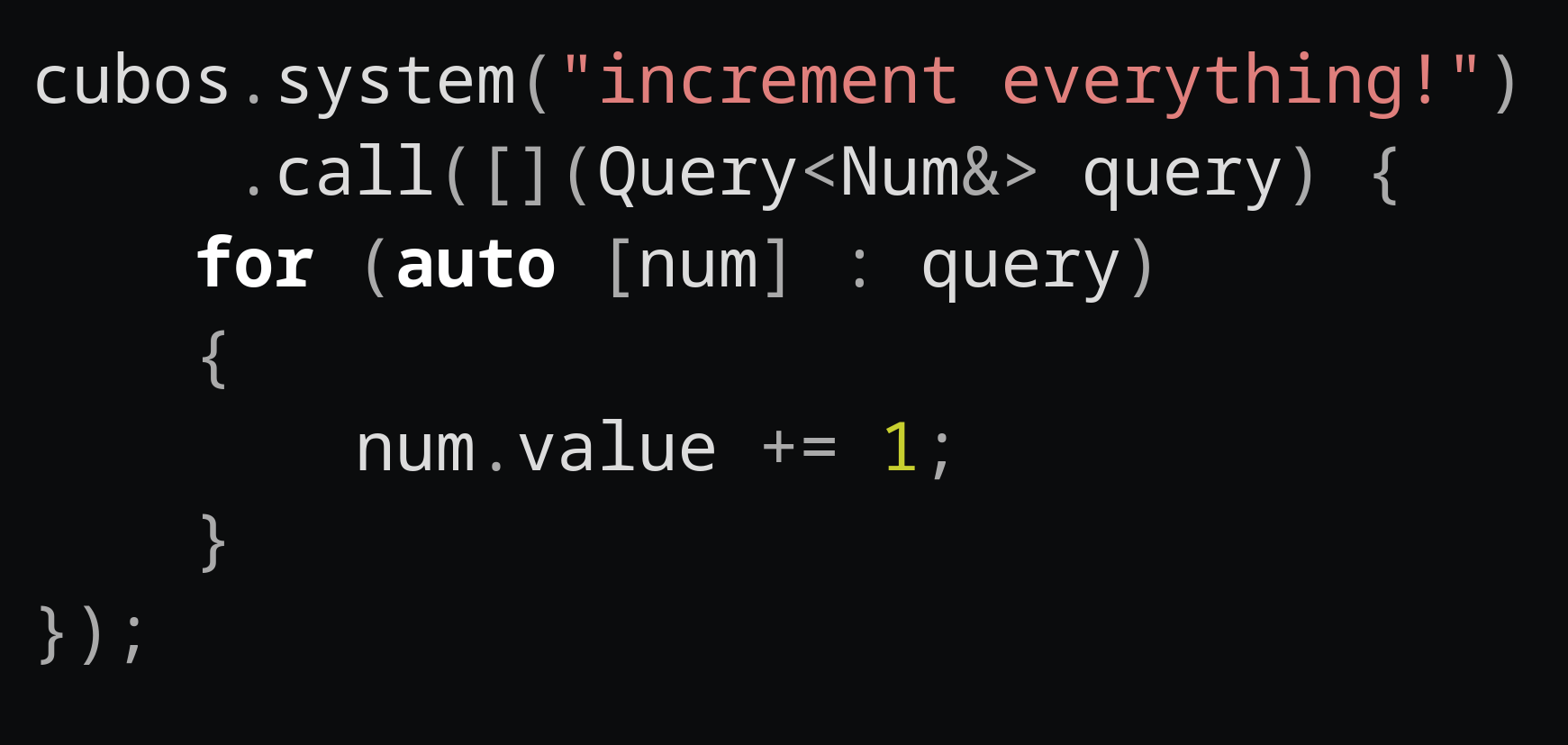
Modular
In Cubos, everything is a plugin: do you prefer to use your own renderer or some other physics framework? Simply swap any of our plugins by your own one.
Physics
Custom physics and collisions engine built specifically for ECS and voxel games.
Audio
Sounds can be easily played from audio assets by creating Audio Source entities.
UI Framework
A custom UI framework built on top of our ECS framework where every element can be manipulated in the same way as any other entity.
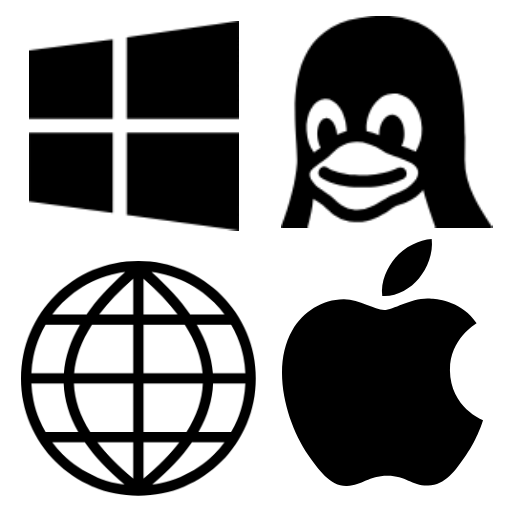
Cross Platform
Support for all major desktop platforms: Windows, MacOS, Linux and Web.
Free and Open Source
The engine and its demos are licensed under the permissive MIT license, which means anyone is free to use, modify and distribute it.
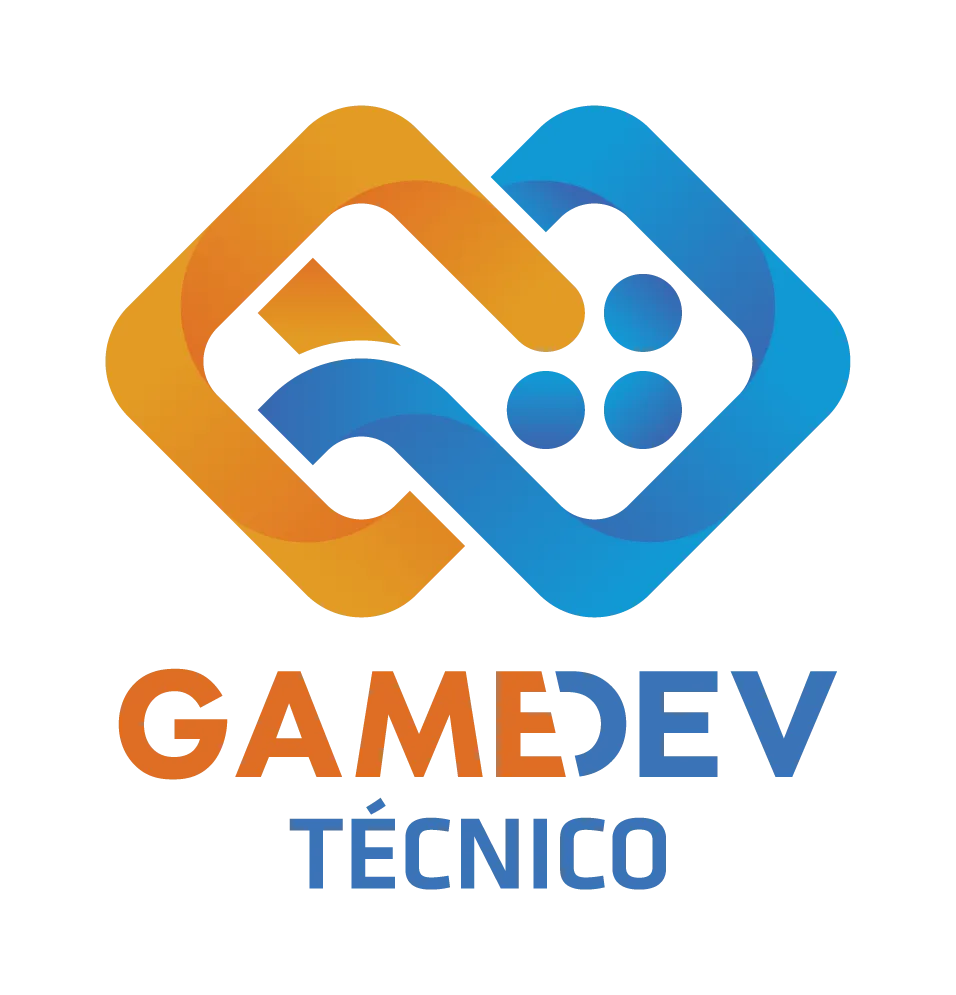
Built by Students
Cubos is developed by students at GameDev Técnico, a student association based at Instituto Superior Técnico, Lisbon, and open to anyone studying in Portugal.
Join us
Are you interested in helping build a game engine from the ground up? Do you want to hone your programming skills and get practical experience on a real C++ codebase? Or, perhaps, would you like to help manage the project, the community, or even improve this website?
Join the Cubos Discord server, where we have more information about you can join the team!